Turbo Pascal, although it is not a world-famous programming application, but the creators who are taking the first steps in writing software begin to get acquainted with this particular environment. It gives the concept of branches, operators, functions and procedures, as well as many other things. For example, when learning, a programmer will encounter cycles in Turbo Pascal: While, For, and Repeat.
The concept of the cycle and its variants
A cycle is called repeating actions. In this environment are used:
- with the parameter (For ... to ... do);
- with a precondition (While ... do);
- with a postcondition (Repeat ... until).
The first type is used when it is known in advance how many steps are in solving the problem. However, there are a number of tasks when there is no information about how many times certain actions will be repeated. In this case, in Pascal While the cycle becomes indispensable, as, in principle, Repeat.
Cycle structure
What is the essence of working in Pascal While, For and Repeat loops? In such constructions, the header and body are distinguished. The first component indicates the variables that will βworkβ, sets the conditions for verifying the truth, the period until which the body will be executed. The second component prescribes the expressions that should be used if the condition is met, i.e. True, not False.
When iteration is performed on the last line of code, then it returns to the header where the condition is checked. In case of truth, the operations are repeated, and if the condition is not met, the program βexitsβ from the cycle and performs further operations.
The following loop looks like this. Pascal ABC and similar programs require writing such code:
- While Condition do;
- Begin
- Body of the cycle;
- End.
If 1 operator (1 action) will be executed in the loop body, then the β...β parentheses begin ... end can be omitted.
Cycle block diagram
The Turbo Pascal While has the following features:
- difficult conditions can be used inside the structure;
- there should not be a semicolon after the word do (this is considered an error in Turbo Pascal and Pascal ABC);
- a variable, constant or expression, which, when receiving a False response, is the output of their subroutine, must be of a logical type, i.e., Boolean.
The following is a block diagram of this kind of cycle. It shows the sequence of actions.
Loop operation algorithm
In the simplest programming environments, including Pascal ABC, While, the cycle operates according to the following principle:
- specified iterations, that is, repetitions, will take place so many times until the condition is true (True);
- as soon as the condition is not satisfied and gives the answer False (or else βFalseβ), the operator exits the loop;
- as soon as this happened, the program βwentβ to the construction, standing after the cycle.
This is a significant difference between While and Repeat, i.e., a cycle with a precondition from a postcondition.
It is very important to provide in the body of the loop the final change of the given variable in the While header. In any case, there should someday be a situation giving a value of False. Otherwise, a loop will occur, and then you will have to use additional measures to exit the compiler. Such errors are considered gross and unforgivable.
How to exit the program during a loop?
Often there is a situation when the While Pascal operator produces a loop in the written program code. What does this mean? The iteration is repeated an infinite number of times, since the condition is always true. For example, here is a fragment of a program:
In this case, to interrupt the task, just press CTRL + F2.
There are 2 more ways to control this behavior of the program. For example, if you write Continue in the code, which transfers control to the beginning of the loop structure (here the condition for exiting the loop is controlled, i.e. the current iteration will be interrupted). Then control is passed in the While loop to the previous check.
The Break statement is able to interrupt the execution of the entire cycle and transfer control to the next iteration. Here the exit from the structure will not be controlled. The image shows examples of using these operators.
Problem solving
Consider the While loop in action. Pascal offers to solve the most diverse problems. Let us dwell on the simplest ones in order to understand the principle of work. Solved tasks in the program Pascal ABC. But images of the classic Turbo Pascal environment will also be presented for comparison.
Task 1: given the function Y = 5-X ^ 2/2. Create a table of values ββin increments of sh = 0.5 over the interval [-5; 5].
Algorithm of actions:
- set the variable X to an initial value of -5 (i.e., the beginning of the interval);
- calculate the value of Y until the variable x reaches the end of the specified segment;
- display the values ββof the function and the abscissas (X);
- increase X by a given step.
This is how the code in Pascal ABC looks.
What the code looks like in Turbo Pascal. The image below illustrates this.
Task 2: given array A, consisting of positive and negative integers. It contains 10 elements. It is necessary to form a matrix B in which the positive elements of array A having an even index will be displayed. Display the sum of the squares in the number from the new matrix.
Algorithm of actions:
- It is necessary to write a subroutine that will "work" only with elements of array A having an even index. In the loop, the value of the variable responsible for the parity of the index will increase by 2.
- If a number with an even index from matrix A corresponds to the condition x> 0, then the counter of the elements of the array increases by 1. The current value of the counter variable will be the index of the number to be copied in array B.
- Initially, the variable summa, which is responsible for finding the sum of squares of positive numbers, is assigned 0. Then the operation will be performed: a new square value will be added to the previous sum.
- Do not be scared if not all positive numbers have passed from one matrix to another. You need to be careful. Many novice programmers rewrite code in a panic. The condition should be carefully studied: positive numbers that are in even "places", that is, having indices that are multiples of 2.
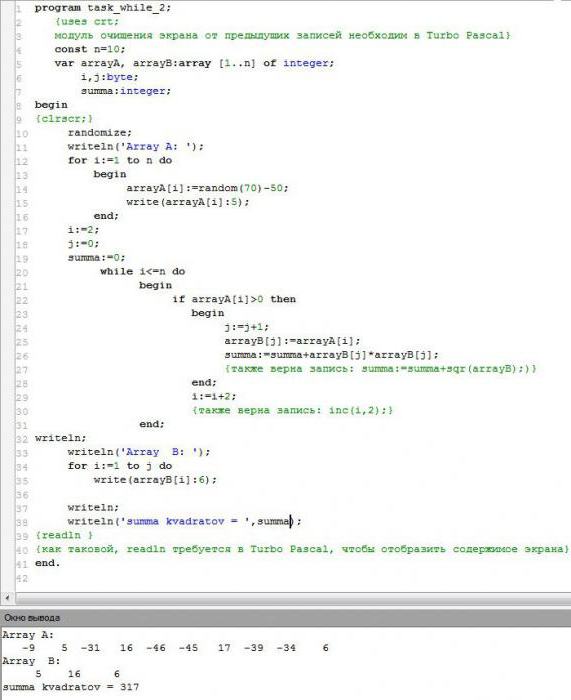
Manual tracing is necessary in order to verify the accuracy of the calculations. Sometimes using this method you can identify errors that do not catch the eye during a routine check of the written code.
If you carry out manual calculations, you can make sure that the program works correctly. This, in turn, suggests that the algorithm for creating the code is correct, the sequence of actions leads to a logical end.