In everyday life, the programmer needs to solve a lot of problems. For these purposes, the Python language is ideal ("Python" or "Python", in the Russian manner). Backing up, creating a game for Android or Windows, reading and saving emails from e-mails are not the most difficult tasks for an experienced programmer. But for beginners, learning Python (working with strings, lists, files) will seem like a fairy tale, since the methods and functions of each data type make life easier for the user.
What is a string data type?
"Python" allows you to work with numbers, symbols, files, functions. And difficulties do not arise anywhere, because this language is convenient and easy to use. Strings in Python are a data type that allows you to process textual information. The length of the entered text can be limited only by computer resources. Keep in mind that strings are an immutable data type. Everything that happens with the original text is assigned to a new variable.
In Python, learning involves learning 2 varieties of text: regular strings (a sequence of bytes) and Unicode-string (a list of characters).
In Python, scripts allow you to use string literals. These characters can be written using the apostrophe, quotation marks (single or double), the specified characters entered three times, for example, "" "apple \ ntree" "", where \ n acts as Enter when typing in a regular text editor. A backslash (backslash) inside literals takes on special meaning. It is required to enter special characters. It should be remembered that the backslash should not be the last character in the program line, otherwise the compiler will throw an error.
How to create a string?
In Python, working with strings involves either manually entering text in the program, or prompting the user to enter the necessary characters. Python reads the received data using the standard input () function. Strings in Python consist of a sequence of characters. You can find out their number using the len () function. In Python, a character is treated as a whole string whose length is 1.
Any object in Python can be converted to a string data type by calling the built-in str () function. There are several classes in Python. To translate an object from one data type to another, you can use the cast function, the name of which coincides with the name of the category. So, int translates to an integer, str to a string, float to a real digit.
Slices in Rows
Otherwise, they are called slices or slices. A slice allows you to extract a character in the specified range from the entered string. Slice cannot be used without indexing. Addressing starts at 0. If you access the line from the end, then indexing begins with the - sign.
ST string | W | O | R | L | D |
Indexing | ST [0] | ST [1] | ST [2] | ST [3] | ST [4] |
Indexing | ST [-5] | ST [-4] | ST [-3] | ST [-2] | ST [-1] |
There are 3 forms of slices:
- Extracting one character from the entire string St [i], where St is the text, i is the cell number.
- Getting a passage of text, St [a: b], where St is the text, a and b are the beginning and end of the gap. A is included in the slice, parameter b is not. If you do not specify b in the interval, but put a colon, the program will return a passage taken to the end of the line. If you do not put down parameter a, then the slice originates from index 0 to the final point b.
- The slice St [a: b: d] allows you to select a specific passage between the beginning at a and the end at b in steps of d.
String Operations
The user has the ability to use string functions in Python:
- Str (X) - translation of any object into a string data type.
- ST1 + ST2 - concatenation (addition of lines).
- ST * n - repeat the ST line n times.
- Min [ST] - returns the minimum value from the code table.
- Len () - determine the length of the text.
- Max [ST] - getting the maximum value from the ASCII table.
- St1 in St2 - the occurrence of the substring St1 in St2. Will return True if St1 is present in the source text.
- St1 not in St2 - Checks for the absence of St1 in St2 and returns True if the statement is true.
String Data Type Methods
In Python, working with strings involves using several methods:
- St.find and St.rfind are the methods needed to find the necessary passage in the entered text. Their difference is that this process will begin from different ends. So, St.find searches from the beginning, and St.rfind from the end. Methods return the index of the first occurrence of the searched passage. You can specify slices to search in a specific interval.
- St.replace (a_old, b_new) is required to replace all occurrences of a substring in the entered text. Instead of a_old there will be b_new. If you specify an additional parameter count (St.replace (a_old, b_new, count)), then the replacement will occur the number of times, no more than count.
- St.count is the method needed to count the number of occurrences of substring S in the entered text. The number of intersections is not included in the final result. You can specify the interval at which the operation will take place.
- St.join is needed to concatenate a list of words.
- St.split is a method that allows you to turn a string into a list of words. In parentheses, a separator is indicated in quotation marks. Most often, this is the space St.split (ββ).
- St.strip - remove spaces at the beginning and end of a line.
- St.capitalize makes the first character in the text capitalized.
- St.swapcase allows you to translate the case of letters in the opposite.
- St.upper makes it possible to capitalize all letters in a string.
- St.lower allows you to translate the entered text in lowercase. All letters will be lowercase.
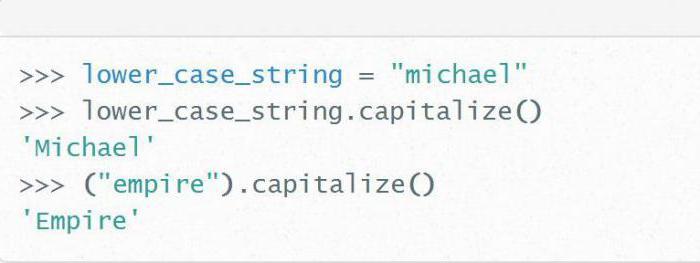
Format string
In Python, working with strings is also pleasant because the user is given the opportunity to format the text according to the specified similarity. That is, the programmer sets the condition to which the entered passage must correspond. Similarly, you can display tables without connecting a special PTable module. This technique is called a format string. For example, if you enter in IDLE print "% s is% d"% ("one", 1) , the result is one is 1 .
% allows you to connect a format string and is its main operator. Python has characters that come in addition to%.
Format character | Explanation |
%from | Single character output. |
% s | String expression. |
% d | Specifies a decimal integer. |
% f | The output of the real (fractional) decimal number. |
There are other special characters, however, they are used in solving complex tasks.
Several tasks with solutions
A language like Python makes learning faster and easier, as the program code is concise and comprehensive. To verify this, you need to parse several tasks.
1. Given a line. Get a new text in which all occurrences of the first β$β character are replaced, except for himself.
Algorithm:
- Write a function in which:
- char is assigned the first character in the string;
- length determines the length of the string;
- str1 through the replace method replaces char with the β$β character;
- str1 writes the first character and the resulting expression from the previous operation;
- return the value of str1.
- Display the result of function calculation.
2. The line is given. Write a code in which βingβ will be added to the end of the word if the word length is> 2. If the word ends with βingβ, then add βlyβ to the line. If the length is less than 2, leave the text unchanged.
Algorithm:
- Write a function in which:
- text length is determined;
- the condition is checked: if the length is> 2 and if the word ends with βingβ, then add βlyβ to such a line, otherwise add βingβ;
- return the value of the resulting variable.
- Display the results of a function with several lines.
To consolidate the result of writing code in Python, it is necessary to solve several more problems with strings.