JQuery is a library of methods written in JavaScript that are designed to simplify working with HTML page elements. This library also greatly simplifies the selection of these elements, because it supports a set of selectors, most of which are borrowed from CSS. In this article, we will talk in detail about all jQuery selectors and give examples of their use.
CSS and jQuery
As mentioned above, selectors in jQuery are borrowed from CSS, however there are a few βBUTsβ here.
- Firstly, jQuery only supports selectors that select DOM elements, i.e. you cannot work with event selectors, for example, hover, and pseudo-elements of first-line.
- Secondly, jQuery has many other selectors that you have not seen in CSS rules, which is why if you already know all the CSS elements, this article will still be relevant for you.
Basic elements
These selectors are used most often, because this is the easiest and most reliable way to select elements. This group is fully consistent with CSS selectors:
Selector | Selector description |
$ ("*") | All elements on the page fall into the selection |
$ ("b") | Elements with the selected tag from the HTML markup fall into the selection, in this example, the <b> ... </b> elements |
$ (". classA") | Elements with the specified class fall into the selection (<div class = "classA">) |
$ ("# IDone") | Elements with the specified id (<div id = "IDone">) fall into the selection |
As in CSS, you can select multiple jQuery selectors at the same time. They can be written with a comma. For example, use the jquery selector by tag name and by ID - $ ("# IDone, b"). It is also allowed to select through a tag + class or a tag + ID, for example, $ ("b.classA").
Attribute selectors
Using various CMS, you may encounter a situation where it is not possible for an HTML markup element to specify an Id or class. The same problem occurs when processing user-generated content. This raises the problem of selecting specific elements, but it is easy to solve using the attribute selector from jquery.
Selector | Selector description |
$ ("div [attribute]") | Processes all elements with the specified attribute, while its value is not taken into account |
$ ("div [attribute = 'value']") | Choosing an element takes into account its attribute and value |
$ ("div [attribute! = 'value']") | Selects the element for which the specified attribute has a value different from the specified. Elements that do not have this attribute also fall into the selection. |
$ ("div [attribute ^ = 'value']") | Selects an element for which the specified attribute begins with the string specified in value |
$ ("div [attribute $ = 'value']") | Selects an element whose specified attribute ends with the line specified in value |
$ ("div [attribute * = 'value']") | Selects an element for which the specified attribute contains in any part the string specified in value |
$ ("div [attribute ~ = 'value']") | Selects an element for which the specified attribute has the word specified in value (a sequence of characters without spaces) |
$ ("div [attribute | = 'value']") | Selects an element for which the specified attribute matches the value specified in value, or begins with it followed by a hyphen |
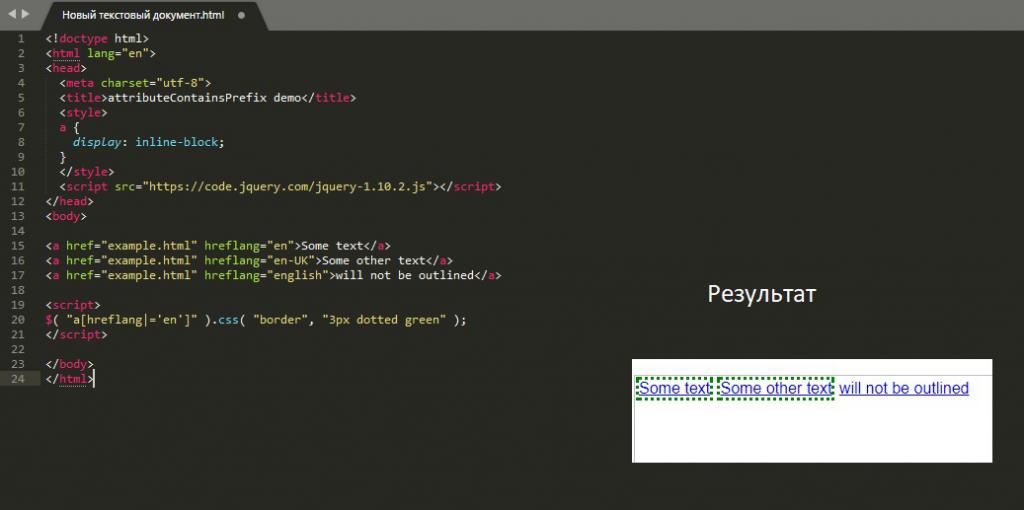
You can combine attributes to narrow the search for matching items, for example, $ ("img [width = 500] [height = 260]").
Selection of elements by content
Rummaging through the contents of HTML page elements and selecting the desired one from the results is a unique jQuery feature. Using this method, you can, for example, make a jquery selector based on the text contained in the paragraph (<p> text </p>).
Selector | Selector example | Selector description |
: contains () | $ ("p: contains ('value')") - selects all paragraphs <p> containing the string 'value'. | Selects the element that contains the specified string. An element will be satisfactory even if the specified string is inside its child element. Remember that this selector is case sensitive, so the string "text" will not match the specified value "TEXT" |
: has () | $ ("p: has (b)") - selects all <p> elements containing <b>. | Selects an element that contains another element indicated in parentheses. This selector also considers child elements. |
: parent | $ ("p: parent") - selects all <p> containing anything. | Selects an item that contains something. |
: empty | $ ("p: empty") - selects all empty <p>. | Selects an item that contains nothing. |
Each of the presented selectors will select a specific element from the code in the image below.
Such a jquery selector can also be combined with others, for example, $ ("p.mail: contains ('e-mail')") will select all paragraphs with the class "mail" that contain the string "e-mail".
Select items by hierarchy
This method is absolutely identical to CSS selectors. It allows you to select elements, depending on their position relative to related elements in the DOM structure. Here itβs better to take apart the jquery selectors with examples.
Selector example | Selector description |
$ ("ul> li") | Selects all elements with the <li> tag that are direct descendants (children) of <ul> |
$ ("ul a") | Selects all elements with the <a> tag that are descendants of any level for <ul> |
$ ("h1 + p") | Selects a sibling with the <p> tag immediately following <h1> |
$ ("li ~ a") | Selects an element with the tag <a>, which immediately follows the <li>, however here they may not be fraternal, but must have a common ancestor |
$ ("li: first-child") | Selects an element with the tag <li>, which is the first child of its parent, for example, <ul> |
$ ("li: last-child") | Selects an element with the tag <li>, which is the last child of its parent, for example, <ul> |
$ ("li: nth-child (3)") | Selects an element with the <li> tag, which is the third child of its parent. Instead of a triple, of course, you can use any other number |
$ ("li: only-child") | Selects those elements with the <li> tag whose parent has only direct descendants (children) |
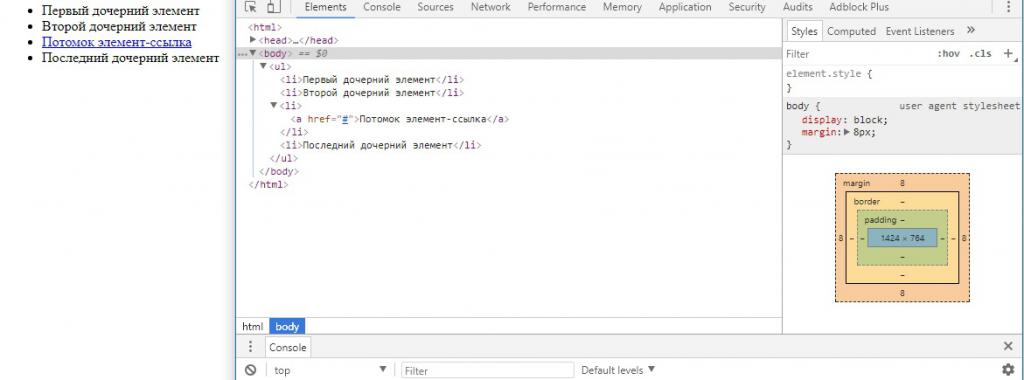
We should also talk about the jquery selector li: nth-child (n), because it allows you to specify not only specific numbers. So he can select all even elements if, instead of n, you set the even constant, or odd, by setting odd. Instead of n, you can also use an expression, for example, $ ("li: nth-child (2n + 3)") will select every second element, starting its count from the third direct child.
Work with form fields
The input tag has many different variations, the operation of which depends on the type attribute. JQuery provides special selectors to select various types of input fields .
Selector | Selector example | Selector description |
: button | $ ("input: button") | Selects all buttons. |
: checkbox | $ ("input: checkbox") | Checkboxes |
: file | $ ("input: file") | File Upload Fields |
: image | $ ("input: image") | Image Input Fields |
: password | $ ("input: password") | Password Fields |
: radio | $ ("input: radio") | Radio buttons |
: reset | $ ("input: reset") | Reset Buttons |
: submit | $ ("input: submit") | Form Submit Buttons |
: text | $ ("input: text") | Fields for text |
: input | $ (": input") | All form fields |
: checked | $ ("input: checked") | Marked fields in checkboxes or radio buttons |
: selected | $ ("option: selected") | Option menu items |
: disabled | $ ("input: disabled") | Disabled form fields |
: enabled | $ ("input: enabled") | Form fields included |
Position selection
The jQuery position selector is very similar to the hierarchy selector. He selects an element according to its position from the list of elements matching the previous condition.
Selector | Description |
: first | Works with the first item from a suitable list |
: last | With the last item from the list |
: eq (n) | Selects an item from the list by its index (n). Attention! The numbering of elements matching the jQuery select condition is from 0! |
: lt () | Selects all items from the list that are before the item with index n |
: gt () | Selects all elements from the list that are after the element with index n |
: even | Items with an even index number are selected |
: odd | Items with an odd index number are selected |
Other selectors
These selectors cannot be attached to any group, but they are no less important. For example, the: not () selector, which can be called logical, allows you to "reverse" a condition or part of it by making a selection from inappropriate conditions.
The selector: hidden will also be equally useful, which will allow you to expand the image element with dimensions 0x0 px to the whole screen, for example, by pressing a button.
Selector | Description |
: not () | Selects items that do not match the condition specified in brackets. |
: animated | Selects the elements that jQuery is currently animating. |
: hidden | Selects elements with the display: none, type = "hidden" property, and with a height and width of 0px. It also applies to elements that contain hidden elements in one of the above ways. Attention! An element with the visibility property set to "hidden" will not fall into the jquery select |
: visible | Reverse: hidden |
: header | Selects the elements h1, h2, h3, h4, h5 and h6 |
What is the best way to use selectors?
Website optimization is an important task, because the load on the server, the userβs convenience, as well as the response time of the interface depend on the quality of its implementation. A lot of books have been written about optimizing scripts in jQuery and JavaScript in general, but they are beyond the scope of this article. We will only give some simple tips that will significantly increase the performance of scripts when fetching elements.
- Try to get around the basic jQuery selectors.
- When choosing several elements for processing, try to combine them into a certain group, and not select each individually. This can be done through the class, or using more specific selectors.
- Using position selection, try to minimize the list from which the selection will occur - this will significantly reduce the speed of searching for the desired item.
An example script with jQuery and its selectors:
Conclusion
Now you know absolutely all selectors from jQuery, in addition, we hope that the examples helped you understand how to build the desired condition from several different selectors.