The main idea of the abstract class lies in the following thesis: sometimes not finished classes are required, but in a "raw" form. Such blanks cannot be directly used (create instances).
What is an abstract class in Java
Consider another example. Java has an abstract Calendar class in the Java.util package. It does not implement a specific calendar, which is used, for example, in Western and Eastern Europe, China, North Korea, Thailand, etc. But it has many useful functions, for example, adding several days to a specific date: these functions are required for any calendar implementation. You cannot spawn an instance from an abstract class.
Abstract classes, abstract Java methods
Suppose you need to develop several graphic elements, for example, geometric shapes: a circle, a rectangle, a star, etc. And there is a container that draws them. Each component has a different appearance, so the corresponding method (let it be called paint) is implemented differently. However, each component has many common features: shapes must be inscribed in a rectangle, can have color, be visible and invisible, etc. That is, you need to create a parent class for all these shapes, where each component will inherit common properties.
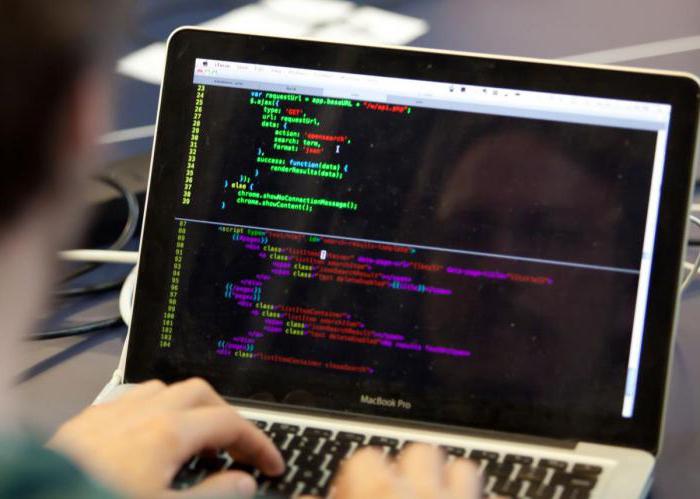
But what about the paint method? After all, the parent class does not have a visual representation. If you declare the paint method in each class independently, you will need to analyze which component is being processed and then type cast. Therefore, the method must be declared abstract in the parent class: set the method title without a body. And the body of each derived class will describe its own. In Java, an abstract class can enclose abstract methods.
If a class has abstract methods, then the class is abstract. The word abstract is preceded by the keyword abstract, and in the method header. After the heading of such a method, you need to put a semicolon. In Java, an abstract class cannot spawn instances. If we want to prohibit their creation, even if the class does not have abstract methods, then the class can be declared abstract. But if a class has at least one abstract method, then the class must be abstract. The class cannot be abstract, and final, and the method either. The method cannot be abstract, private, static, native. In order for descendant classes to be declared non-abstract and to instantiate them, they must implement all the abstract methods of the parent. The class itself can use its abstract methods.
Example:
- abstract class AClass {
- public abstract void method (int a);
- }
- class BClass extends AClass {
- public void method (int a) {
- // body
- }
Variables of the type abstract class are allowed. They can refer to a non-abstract descendant of this class or be null.
Interfaces in Java - An Alternative to Multiple Inheritance
There is no multiple inheritance in Java, because then certain problems arise. A class cannot inherit from multiple classes. But then he can implement several interfaces.
Interfaces and abstract Java classes are concepts that are similar, but not the same. The interface can be declared as public, then it is accessible to everyone, or you can omit the public modifier, then the interface is available only inside its package. The abstract keyword is not required, because the interface is already abstract, but you can specify it.
Interface Announcement
It starts with a header and the public keyword can go first, then the interface word. Then the word extends and an enumeration of the interfaces from which this one is inherited can go. It does not allow repetition, and it is also impossible for the inheritance relation to form a cyclical dependence. Then comes the body of the interface, enclosed in curly braces. Elements are declared in the body of the interface: constant fields and abstract methods. All fields are public final static - all of these modifiers are optional. All methods are considered public abstract - these modifiers can also be specified. Now quite enough has been said about the difference between an abstract class and the Java interface.
- public interface AI extends B, C, D {
- // body
- }
To declare a class as an inheritor of an interface, use the implements keyword:
- class AClass implements BI, CI, DI {}
That is, if the interface name is indicated after implements in the class declaration, then the class implements it. The heirs of this class get its elements, so they also implement it.
Interface type variables are also allowed. They can refer to the type of the class that implements this interface, or null. Such variables have all the elements of the Object class, because objects are derived from classes, and they, in turn, are inherited from the Object class.
In this article, we looked at some elements of the Java object model — abstract classes, abstract methods, and interfaces.